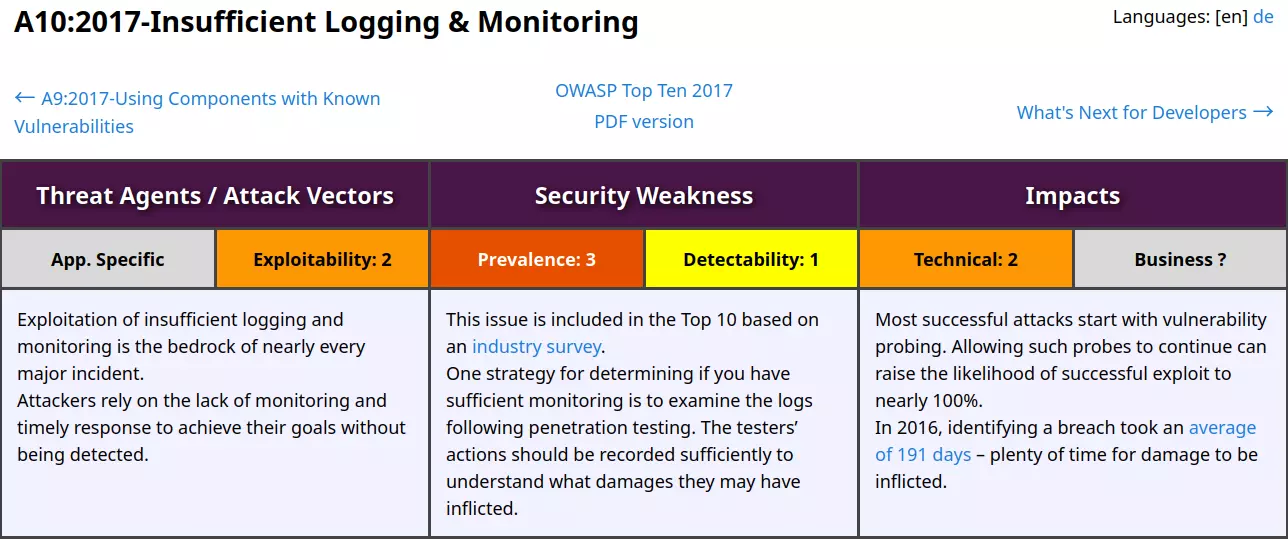
OWASP A10:2017 – Insufficient Logging & Monitoring
If you stumble across this post and are wondering what this is all about, then I recommend reading this post before following this guide. TL; DR, this post is about solving Secure Code Warrior challenges, more specifically their PHP Basic challenges. So, without further ado, let’s get started.
Table of Contents
Challenge 1 – Insufficient Logging and Monitoring
Locating Vulnerability
As the challenge name suggests, we should be looking for vulnerable code that doesn’t implement proper logging to events the application goes through. As such, the writeLog() function of Logger.php is vulnerable as it doesn’t log enough data(almost nothing) that’d prove useful to detect an attacker.
/**
* @param string $message
*/
public static function writeLog(string $message): void
{
echo "$message\n";
}
In the above code the logging function will only log the messages predefined in the application source code to a log file and would not log important events like error events, security events, app crashes etc.
Identifying Solution
There are many ways to approach logging. But OWASP suggests to always log the following:-
- Input validation failures e.g. protocol violations, unacceptable encodings, invalid parameter names and values
- Output validation failures e.g. database record set mismatch, invalid data encoding
- Authentication successes and failures
- Authorization (access control) failures
- Session management failures e.g. cookie session identification value modification
- Application errors and system events e.g. syntax and runtime errors, connectivity problems, performance issues, third party service error messages, file system errors, file upload virus detection, configuration changes
- Application and related systems start-ups and shut-downs, and logging initialization (starting, stopping or pausing)
- Use of higher-risk functionality e.g. network connections, addition or deletion of users, changes to privileges, assigning users to tokens, adding or deleting tokens, use of systems administrative privileges, access by application administrators,all actions by users with administrative privileges, access to payment cardholder data, use of data encrypting keys, key changes, creation and deletion of system-level objects, data import and export including screen-based reports, submission of user-generated content – especially file uploads
- Legal and other opt-ins e.g. permissions for mobile phone capabilities, terms of use, terms & conditions, personal data usage consent, permission to receive marketing communications
Also, before creating your own php logger class try to refer pre-written and well documented logger classes that can be found online. For e.g., there are many php logger projects on Github and I found this Simple-PHP-Logger good enough to recommend having a read. As such, our desired secure code solution needs to implement multiple levels of logging, i.e., alert, critical, error, warning, info etc. and should include all user events/actions taken in the application. The logger class must implement all levels of logging for getting detailed messages on user events/actions and only on solution implements it properly.
<?php
namespace App\services\logger;
use App\config\Config;
use App\services\singleton\Singleton;
use DateTime;
use Framework\HTTP\Session;
class Logger extends Singleton
{
/**
* @var Singleton
*/
private static Singleton $logger;
/**
* @var false|resource
*/
private static $fileHandle;
private const ALERT = 'ALERT';
private const CRITICAL = 'CRITICAL';
private const ERROR = 'ERROR';
private const WARNING = 'WARNING';
private const INFO = 'INFO';
private function __construct()
{
parent::__construct();
$config = Config::getConfig();
self::$logger = static::getInstance();
self::$fileHandle = fopen($config['log_path'], 'wb');
}
/**
* @param string $emergencyLevel
* @param string $message
*/
public static function writeLog(string $emergencyLevel, string $message): void
{
$dateTime = new DateTime();
$date = $dateTime->format('Y-m-d');
$user = Session::getUserToken();
fwrite(self::$fileHandle, "[$date] - $emergencyLevel: $user $message\n");
fclose(self::$fileHandle);
}
/**
* @param string $message
*/
public static function alert(string $message): void
{
self::writeLog(self::ALERT, $message);
}
/**
* @param string $message
*/
public static function critical(string $message): void
{
self::writeLog(self::CRITICAL, $message);
}
/**
* @param $message
*/
public static function error($message): void
{
self::writeLog(self::ERROR, $message);
}
/**
* @param $message
*/
public static function warning($message): void
{
self::writeLog(self::WARNING, $message);
}
/**
* @param $message
*/
public static function info($message):void
{
self::writeLog(self::INFO, $message);
}
}
Challenge 2 – Insufficient Logging and Monitoring
Locating Vulnerability
The solution is same as Challenge 1.
Identifying Solution
The solution is same as Challenge 1.
Challenge 3 – Insufficient Logging and Monitoring
This challenge is also same as the other two challenges and as such the solutions are same.
Conclusion
This category concludes the OWASP TOP 10 2017 series of vulnerabilities. I hope this helps me find security bugs and CVEs in open source projects. 🙂
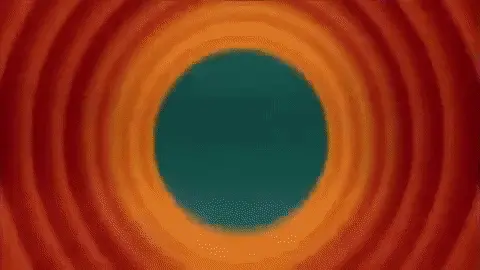