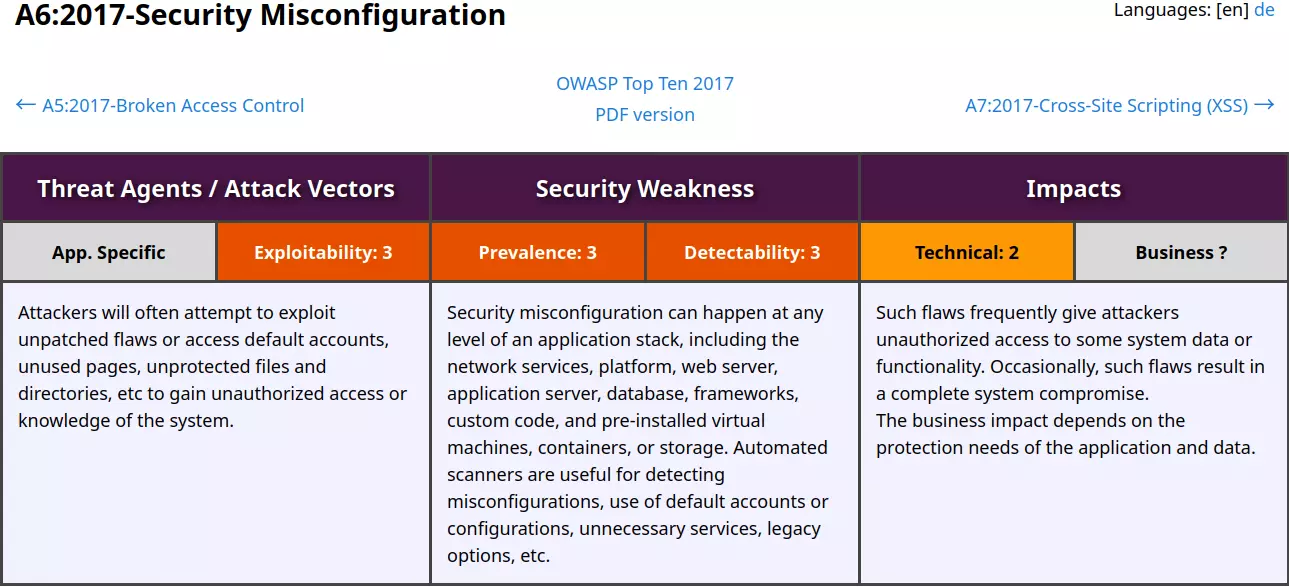
OWASP A6:2017 – Security Misconfiguration
If you stumble across this post and are wondering what this is all about, then I recommend reading this post before following this guide. TL; DR, this post is about solving Secure Code Warrior challenges, more specifically their PHP Basic challenges. So, without further ado, let’s get started.
Table of Contents
Challenge 1 – Debug Features Enabled
Locating Vulnerability
As the challenge is about unnecessary debug features being enabled, we should look for implementation in the code where debug is enabled or can be enabled via custom HTTP requests. As such, the archive_reports.php file in /views/cabinet/ has set debug to be enabled via a request parameter.
if ($_GET['debug'] === 'Y') {
print_r((new PersonalCabinetController($request))->getReports(null, true, $_GET['id']));
}
Enabling debug features in development environments can be useful for troubleshooting problems in code, but allowing debug in production can provide useful information to an attacker to mount further attacks. For e.g., based on the above code an attacker can craft a curl command like the following to get useful information out of the application:-
curl http://example.com/archive_reports.php?id=4345&debug=1
As you can see from the above command, the attacker is able to enable debug mode by just adding a parameter to the HTTP GET request and in return was able to dump all reports via the getReports() function.
Identifying Solution
The best solution to the above problem is to get rid of every debug feature implemented throughout the application code. Debug parameters like the one above should never be shipped into production which will prevent an attacker from getting access to sensitive information. The solution is pretty obvious and as such I’m not posting the solution code here.
Challenge 2 – Disabled Security Features
Locating Vulnerability
As the challenge name suggests, we need to look for security features that are disabled and as a result pose a security risk for the application. As such, if you look inside the php.ini PHP configuration file you’ll see that httponly cookie security flag is disabled.
session.cookie_httponly = false
The httponly cookie flag makes the cookie accessible only over HTTP and prevents client-side scripts like javascript code from accessing the cookie. This prevents cookie stealing via XSS attacks.
Identifying Solution
The solution is super simple just set the configuration to true.:)
session.cookie_httponly = true
Challenge 3 – Disabled Security Features
Locating Vulnerability
In this challenge security headers are explicitly defined in application source code in the HeadersHelper.php file.
<?php
namespace App\helpers;
class HeadersHelper
{
public static function setHeaders()
{
header('Access-Control-Allow-Methods', 'POST, GET');
header('Strict-Transport-Security', 'max-age=315360000');
header('Cache-Control', 'no-cache, no-store');
}
}
One proper way of defining security headers is via Webserver(like Apache, Nginx) configuration files. Explicitly defining some security headers while leaving out others may result in essential security headers being ignored(as they’re not defined) or make the application use their default values. Absence of security headers can lead many types of vulnerabilities in application.
Identifying Solution
Implementing security headers can be a bit trouble if you’re not exactly sure what you’re doing and the sources from where you’re getting information about the headers aren’t reliable. When it comes to Web Application Security nobody does it better than OWASP and as such you can’t find the reference for the solution to this challenge from the OWASP Secure Headers Project where they’ve laid out some best practices for you to follow while implementing Security Headers in your web application. Now, if you’re feeling over enthusiastic and would like contribute to the Secure Headers Project, then you can head over to their github repo of the project. The solution to this challenge is easy and just a little bit tricky. As I’ve provided every resource you needed to solve this challenge, I think I’ll pass this challenge over to the reader to solve. 🙂
Conclusion
Five of OWASP Top 10 2017 categories down, five more to go.:)